Table of Contents

What is HTML?
HTML (HyperText Markup Language) is the standard language used to create and structure content on the web. It provides the framework for web pages, allowing developers to define the structure and layout of text, images, links, multimedia, and other elements.
Key Features of HTML:
- Markup Language: HTML uses “tags” to define the content structure. Tags are enclosed in angle brackets, e.g.,
<html>
,<head>
,<body>
,<p>
, etc. - Elements: An HTML element consists of a start tag, content, and an end tag. For example:
<p>This is a paragraph.</p>
- Attributes: Tags can have attributes to provide additional information or customization. For example:
<a href="https://www.example.com">Visit Example</a>
Here,href
is an attribute that specifies the link destination. - Hierarchical Structure: HTML organizes content in a nested, hierarchical way, starting with the
<html>
element, which contains the<head>
and<body>
sections.
Basic HTML Document Structure:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>Welcome to HTML</h1>
<p>This is a simple HTML page.</p>
</body>
</html>
HTML is the foundation of most web pages and works alongside CSS (for styling) and JavaScript (for interactivity) to create fully functional websites.
What is HTML used for?
HTML (HyperText Markup Language) is primarily used to create and structure content for web pages. It is the backbone of the web and serves several essential purposes:
Primary Uses of HTML:
- Structuring Web Pages:
- HTML defines the layout and structure of a web page using various elements such as headings (
<h1>
to<h6>
), paragraphs (<p>
), lists (<ul>
,<ol>
), and divisions (<div>
). - Example:
<h1>Welcome</h1> <p>This is a paragraph on the web page.</p>
- HTML defines the layout and structure of a web page using various elements such as headings (
- Embedding Content:
- It allows the integration of multimedia content like images, videos, and audio into web pages.
- Example:
<img src="image.jpg" alt="Example Image"> <video controls> <source src="video.mp4" type="video/mp4"> </video>
- Creating Hyperlinks:
- HTML enables navigation between different web pages or sections within the same page through hyperlinks.
- Example:
<a href="https://www.example.com">Visit Example</a>
- Interactive Forms:
- It is used to create forms for collecting user data, such as login forms, contact forms, and surveys.
- Example:
<form action="/submit" method="post"> <label for="name">Name:</label> <input type="text" id="name" name="name"> <button type="submit">Submit</button> </form>
- Document Metadata:
- HTML provides metadata (information about the web page) through elements in the
<head>
section, such as the page title, description, and character encoding. - Example:
<meta charset="UTF-8"> <title>My Web Page</title>
- HTML provides metadata (information about the web page) through elements in the
- Accessibility and SEO:
- HTML helps improve accessibility and search engine optimization (SEO) by using semantic elements like
<header>
,<footer>
,<article>
, and<nav>
to provide context about the content.
- HTML helps improve accessibility and search engine optimization (SEO) by using semantic elements like
- Combining with Other Technologies:
- HTML is used in conjunction with:
- CSS (Cascading Style Sheets) for styling and layout.
- JavaScript for interactivity and dynamic content.
- HTML is used in conjunction with:
Why HTML is Important:
- It is universally supported by web browsers.
- It serves as the starting point for web development.
- It ensures compatibility and consistency across the internet.
In short, HTML is essential for building the structural foundation of websites and web applications.
How to Write HTML
How to Write HTML
Writing HTML involves creating a file with .html
extension and using HTML tags to define the structure and content of a web page. Here’s a step-by-step guide:
1. Tools You Need:
- Text Editor: You can use any plain text editor like:
- Notepad (Windows)
- TextEdit (Mac, in plain text mode)
- Or specialized editors like VS Code, Sublime Text, or Atom.
- Web Browser: To view and test your HTML file (e.g., Chrome, Firefox, Safari).
2. Basic Structure of an HTML Document
All HTML documents follow a specific structure:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>Welcome to My Page</h1>
<p>This is a paragraph on my web page.</p>
</body>
</html>
<!DOCTYPE html>
: Declares the document type and version (HTML5 in this case).<html>
: The root element of the HTML document.<head>
: Contains metadata (e.g., title, links to CSS, and scripts).<body>
: Contains the content that will appear on the web page.
3. Save Your File
- Save the file with a
.html
extension (e.g.,index.html
). - Ensure the file encoding is set to UTF-8 (most editors handle this automatically).
4. Open the HTML File
- Double-click the saved
.html
file and it will open in your default web browser. - Alternatively, right-click the file and select “Open With” to choose a specific browser.
5. Start Adding Content
Here are some examples of common HTML elements:
Headings
<h1>Main Heading</h1>
<h2>Subheading</h2>
Paragraphs
<p>This is a paragraph.</p>
Links
<a href="https://www.example.com">Visit Example</a>
Images
<img src="image.jpg" alt="An example image">
Lists
- Ordered List:
<ol> <li>First item</li> <li>Second item</li> </ol>
- Unordered List:
<ul> <li>Item one</li> <li>Item two</li> </ul>
Tables
<table border="1">
<tr>
<th>Name</th>
<th>Age</th>
</tr>
<tr>
<td>John</td>
<td>25</td>
</tr>
</table>
Forms
<form action="/submit" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<button type="submit">Submit</button>
</form>
6. Add Styling (Optional)
You can style your HTML with CSS by adding a <style>
tag in the <head>
or linking to an external CSS file:
<head>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
}
h1 {
color: blue;
}
</style>
</head>
7. Test and Refine
- Open your file in a browser to see the results.
- Modify the file in your editor, save changes, and refresh the browser to see updates.
By following these steps, you can create and enhance web pages with HTML.
Common HTML Elements
Common HTML Elements
HTML uses elements (tags) to structure and display content on a web page. Here’s a list of commonly used HTML elements, organized by their purpose:
1. Structural Elements
These define the basic layout of a web page.
<html>
: The root element of an HTML document.<head>
: Contains metadata, scripts, styles, and the title of the document.<body>
: Contains the content displayed on the web page.
Example:
<!DOCTYPE html>
<html>
<head>
<title>My Web Page</title>
</head>
<body>
<!-- Content goes here -->
</body>
</html>
2. Text Formatting Elements
Used to define headings, paragraphs, and text styles.
- Headings:
<h1>
to<h6>
(largest to smallest)<h1>Heading 1</h1> <h2>Heading 2</h2>
- Paragraphs:
<p>
<p>This is a paragraph.</p>
- Bold and Italic:
<b>
or<strong>
(bold),<i>
or<em>
(italic)<strong>Important text</strong> <em>Emphasized text</em>
- Line Break:
<br>
Line 1<br>Line 2
3. Links and Navigation
Used to create links and navigation elements.
- Anchor (Link):
<a>
<a href="https://www.example.com">Visit Example</a>
- Navigation Bar:
<nav>
<nav> <a href="#home">Home</a> <a href="#about">About</a> </nav>
4. Media Elements
Used to embed images, videos, and audio.
- Image:
<img>
<img src="image.jpg" alt="Example Image">
- Video:
<video>
<video controls> <source src="video.mp4" type="video/mp4"> </video>
- Audio:
<audio>
<audio controls> <source src="audio.mp3" type="audio/mp3"> </audio>
5. List Elements
Used to create ordered and unordered lists.
- Unordered List:
<ul>
with<li>
for items<ul> <li>Item 1</li> <li>Item 2</li> </ul>
- Ordered List:
<ol>
with<li>
for items<ol> <li>First</li> <li>Second</li> </ol>
6. Table Elements
Used to display tabular data.
- Table:
<table>
with<tr>
(row),<th>
(header), and<td>
(cell)<table border="1"> <tr> <th>Name</th> <th>Age</th> </tr> <tr> <td>John</td> <td>25</td> </tr> </table>
7. Form Elements
Used for collecting user input.
- Form:
<form>
with input fields<form action="/submit" method="post"> <label for="name">Name:</label> <input type="text" id="name" name="name"> <button type="submit">Submit</button> </form>
8. Semantic Elements
Provide meaningful structure to the web page.
- Header:
<header>
<header> <h1>Site Title</h1> </header>
- Footer:
<footer>
<footer> <p>© 2024 My Website</p> </footer>
- Section:
<section>
<section> <h2>About Us</h2> <p>Information about us.</p> </section>
- Article:
<article>
<article> <h2>Blog Post</h2> <p>This is a blog post.</p> </article>

9. Inline Elements
Used for small pieces of content within a block.
- Span:
<span>
for styling or grouping text<p>This is <span style="color: red;">important</span>.</p>
- Code:
<code>
for displaying code<code>console.log("Hello, World!");</code>
10. Miscellaneous
- Horizontal Line:
<hr>
<p>Before</p> <hr> <p>After</p>
- Divisions:
<div>
for grouping content<div> <p>Group of content.</p> </div>
These elements form the foundation of HTML and are used to create and style web pages.
Common HTML Attributes
Common HTML Attributes
Attributes in HTML provide additional information about an element and are always included within the opening tag. They are written as key-value pairs (e.g., attribute="value"
).
Here’s a list of commonly used HTML attributes and their purposes:
1. Global Attributes
These attributes can be applied to almost any HTML element.
id
: Defines a unique identifier for an element.<div id="unique-id">Content</div>
class
: Assigns one or more classes to an element for styling or scripting.<p class="highlight">This is highlighted text.</p>
style
: Adds inline CSS styling.<h1 style="color: blue;">Styled Heading</h1>
title
: Provides additional information (tooltip) when the user hovers over the element.<p title="Tooltip text">Hover over this text.</p>
lang
: Specifies the language of the element’s content.<p lang="en">Hello, World!</p>
data-*
: Custom data attributes for embedding custom information.<div data-user-id="12345">User Info</div>
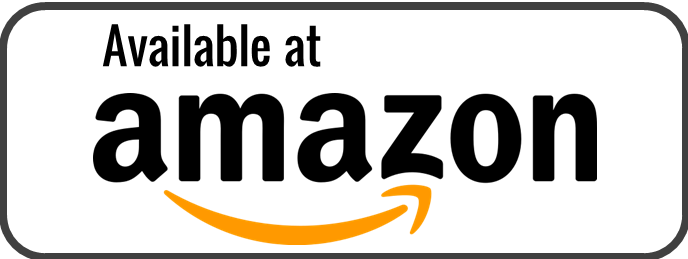
2. Attributes for Hyperlinks (<a>
Tag)
href
: Specifies the URL of the link.<a href="https://www.example.com">Visit Example</a>
target
: Specifies where to open the link. Common values:_self
(default): Opens in the same tab._blank
: Opens in a new tab.
<a href="https://www.example.com" target="_blank">Open in New Tab</a>
rel
: Specifies the relationship between the current document and the linked document.<a href="https://www.example.com" rel="nofollow">No Follow Link</a>
3. Attributes for Images (<img>
Tag)
src
: Specifies the image source (URL or file path).<img src="image.jpg" alt="An example image">
alt
: Provides alternative text for the image (important for accessibility and SEO).<img src="logo.png" alt="Company Logo">
width
andheight
: Sets the image dimensions.<img src="photo.jpg" width="200" height="100">
4. Attributes for Forms and Inputs
action
: URL to which form data is submitted.<form action="/submit" method="post">
method
: HTTP method used for form submission (GET
orPOST
).<form action="/submit" method="get">
name
: Assigns a name to an input for identification.<input type="text" name="username">
placeholder
: Provides placeholder text inside an input field.<input type="text" placeholder="Enter your name">
value
: Sets the default value of an input field.<input type="text" value="Default Text">
required
: Marks an input field as mandatory.<input type="email" required>
disabled
: Disables an input field.<input type="text" disabled>
checked
: Marks a checkbox or radio button as selected by default.<input type="checkbox" checked>
maxlength
: Specifies the maximum number of characters allowed.<input type="text" maxlength="10">
5. Attributes for Tables
colspan
: Merges multiple columns into one.<td colspan="2">Merged Cell</td>
rowspan
: Merges multiple rows into one.<td rowspan="3">Merged Row</td>
border
: Specifies the width of the table border.<table border="1">
6. Media Attributes
controls
: Adds playback controls for audio or video.<video controls> <source src="video.mp4" type="video/mp4"> </video>
autoplay
: Plays the media automatically when the page loads.<audio autoplay> <source src="audio.mp3" type="audio/mp3"> </audio>
loop
: Loops the media playback.<video loop> <source src="video.mp4" type="video/mp4"> </video>
muted
: Mutes the audio or video by default.<video muted> <source src="video.mp4" type="video/mp4"> </video>
7. Event Attributes
These attributes handle JavaScript events like clicks, hover actions, etc.
onclick
: Executes JavaScript when the element is clicked.<button onclick="alert('Button Clicked!')">Click Me</button>
onmouseover
: Executes JavaScript when the mouse hovers over the element.<p onmouseover="this.style.color='red'">Hover over me!</p>
8. Accessibility Attributes
aria-*
: Used to improve accessibility (ARIA = Accessible Rich Internet Applications).<button aria-label="Close">X</button>
9. Deprecated Attributes
Some older attributes are no longer recommended (e.g., <font>
, align
, bgcolor
). Use CSS instead for styling.
Understanding and using these attributes properly can help you create well-structured, accessible, and interactive web pages!
How to Learn HTML
How to Learn HTML
Learning HTML is straightforward, as it’s one of the easiest programming languages to understand. Here’s a guide to help you start and master HTML:
1. Understand the Basics
- What is HTML? Learn that HTML is used to structure web pages.
- Basic Syntax: Understand elements, tags, and attributes.
- Tags:
<tagname>Content</tagname>
- Attributes:
<tagname attribute="value">
- Tags:
Start with simple examples like:
<!DOCTYPE html>
<html>
<head>
<title>My First Webpage</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>Welcome to HTML learning.</p>
</body>
</html>
2. Use Online Tutorials and Resources
Interactive Tutorials:
- W3Schools: Beginner-friendly with examples and an online editor.
- freeCodeCamp: Comprehensive courses for HTML, CSS, and JavaScript.
- Mozilla Developer Network (MDN): Detailed documentation for all HTML elements and attributes.
Video Tutorials:
- Search for “HTML for Beginners” on YouTube. Channels like Traversy Media and The Net Ninja offer excellent content.
3. Practice with Hands-On Projects
The best way to learn HTML is by building projects. Start simple and gradually increase complexity:
- Create a personal profile page.
- Build a static webpage with headings, images, and lists.
- Add a contact form using
<form>
.
4. Experiment with Online Editors
Use online tools to write and test HTML code instantly:
5. Explore Advanced Topics
Once you’ve mastered the basics, move on to advanced topics:
- Semantic HTML: Learn about elements like
<header>
,<footer>
,<section>
, and<article>
. - Forms and Input: Explore advanced input types and form validation.
- Accessibility: Use ARIA roles and attributes to make web pages accessible.
6. Pair HTML with CSS and JavaScript
To build modern and functional web pages:
- Learn CSS for styling: colors, layouts, and animations.
- Learn JavaScript for interactivity: buttons, forms, and dynamic content.
7. Use Challenges and Exercises
Practice with coding challenges to solidify your skills:
- Frontend Mentor
- HackerRank (HTML/CSS projects)
- Codewars
8. Build Real-World Projects
Create and deploy your own projects:
- Portfolio website
- Blog template
- Product landing page
- Static business website
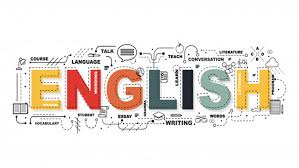
9. Participate in Communities
Join communities where you can ask questions and share your progress:
- Stack Overflow
- Reddit: r/webdev
- Discord or Slack groups for web development.
10. Keep Practicing and Exploring
HTML is constantly evolving (e.g., HTML5). Stay updated by:
- Reading web development blogs.
- Following web standards from W3C.
Suggested Learning Path
- Start with basic tags and attributes.
- Create simple pages with headings, paragraphs, and images.
- Learn lists, tables, and forms.
- Study semantic HTML.
- Build your own website or projects.
With consistent practice, you can master HTML in a few weeks!